Blog
Mastering JAX Arange on Loop Carry for High-Performance Computing
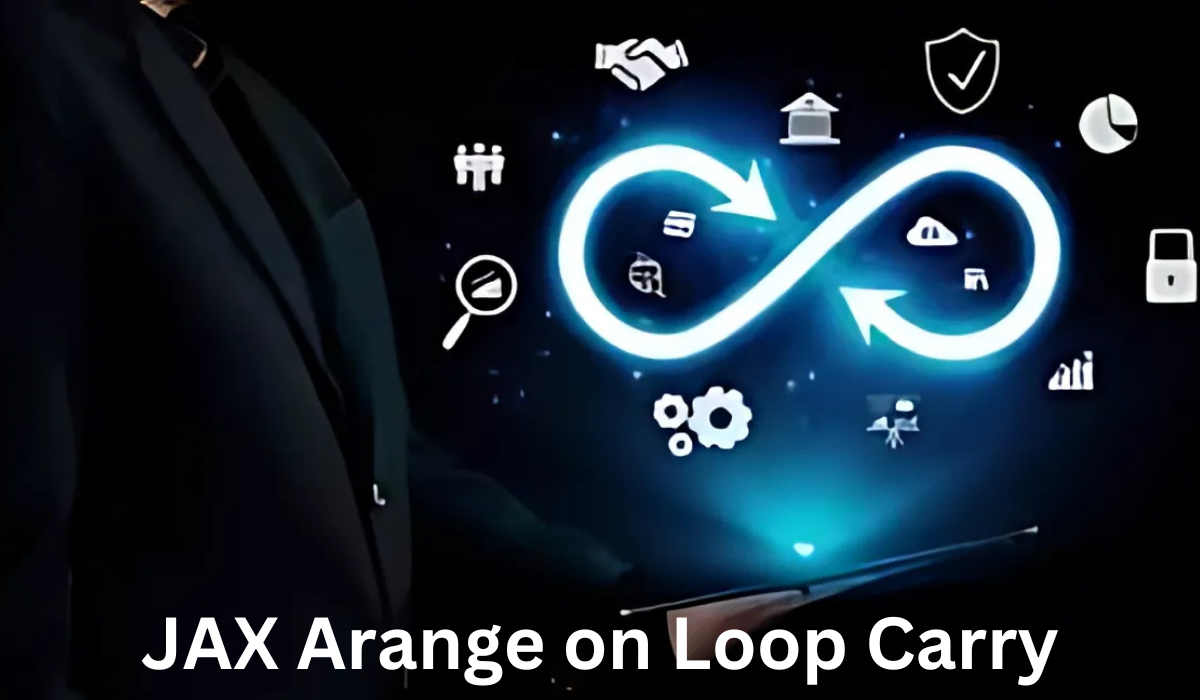
Introduction
Automation and efficiency are at the heart of high-performance computing, especially in data-heavy Python applications. While traditional loops in Python can pose performance bottlenecks, libraries like JAX provide solutions for scalable optimization. If you’re a Python developer or a computing enthusiast eager to bring efficiency to your projects, jax arange on loop carry optimization techniques will elevate your workflow.
This post, Jax arange on loop carry, will guide you through how JAX can supercharge your computational tasks with features like vectorization, automatic differentiation, and parallel execution. By the end of this blog, you’ll know how to harness the power of JAX for optimized loop carries—saving both time and resources.
What is JAX? A Quick Overview
JAX is a Python library designed for high-performance numerical computing. Built on NumPy, JAX enables:
- Automatic differentiation (Autograd) for optimized computations,
- GPU/TPU acceleration for parallel execution and
- Vectorized operations for scaled, loop-free implementations.
For developers working with large datasets, scientific computing, or deep learning, JAX provides the perfect blend of simplicity and power for computations.
Why JAX Matters for Developers
Python’s versatility and ease of use sometimes come at the cost of speed on larger-scale computations. Loops, a Python staple, especially slow down execution when dealing with high-dimensional datasets. JAX resolves this by inherently supporting optimized vectorization and enabling features like Just-In-Time (JIT) compilation.
Key features of JAX include:
- Efficient Array Manipulation with NumPy-like functions (`jax.numpy` methods),
- Parallel Processing across GPUs and TPUs,
- Memory Optimization for seamless execution without lags.
With JAX’s array-centric approach, you can build highly efficient workflows for complex operations like loop carriers.
The Core Power of Jax arange on loop carry Optimization
The `jax.numpy.arange` function shines when dealing with large-scale data preparation in loops. It allows the creation of sequential arrays that form a bedrock for calculations, making it ideal for optimization in operations.
For instance:
- Instead of manually iterating over array elements, the `arange` function lets JAX vectorize these operations.
- This reduces the strain on Python’s Global Interpreter Lock (GIL), streamlining memory access patterns.
Key Benefits of JAX in Loop Carry Optimization
Efficient use of `arange` in combination with JAX’s capabilities ensures:
1. Vectorization
Rather than iterating through elements in a traditional loop, JAX processes entire data arrays simultaneously, which minimizes computational overhead and boosts execution speed.
2. Efficient Memory Access
Loop carry optimizations in JAX eliminate redundant memory calls, providing direct access to sequential data—ideal for GPU and TPU acceleration.
3. Automatic Differentiation
JAX’s autograd simplifies gradient calculations for array operations, which is critical for projects involving machine learning or numerical simulations.
4. Hardware Acceleration
JAX supports built-in parallel execution on GPUs and TPUs, reducing the resources and time required for computation-heavy operations.
Hands-On Demonstration
Here’s an example to showcase how JAX performs optimized loop carries.
Example Code Implementation
“`
import jax
import jax. numpy as np
Sample operation definition
def optimized_function(arr):
return jnp.sin(arr) * jnp.cos(arr)
Create a large-scale dataset using jax.numpy.arrange
arr = jnp.arange(100_000)
Optimize execution with JAX’s Just-In-Time (JIT) compilation.
optimized_result = jax.jit(optimized_function)(arr)
“`
Breakdown of the Code:
1. Array Generation
The array is created using `jump.arange(100_000)`, efficiently preparing the dataset in memory.
2. Vectorized Operations
The `optimized_function` applies element-wise mathematical operations (`sin` and `cos`) across the array.
3. JIT Compilation
The function is compiled at runtime, reducing redundant computation for faster execution.
This approach ensures efficient reuse of memory resources and faster results, which is perfect for handling data-heavy operations in a professional environment.
Performance Metrics
Compared to traditional Python loops, JAX’s vectorized loop carry operations reduce execution time significantly while ensuring lower memory usage on larger data structures. For example, developers working on real-time data analytics can scale seamlessly using such optimizations.
Advantages of JAX for Enterprise and HPC Use Cases
Beyond simple use cases, using Jax arange on loop carry operations extends to enterprise-level computational needs, benefiting the following domains:
1. Data Science and Analytics
- Run large-scale simulations (e.g., Monte Carlo simulations) faster.
- Build optimized models/algorithms without worrying about memory constraints.
2. Machine Learning Pipelines
- Perform gradient-based optimization easily using Autograd.
- Parallelize deep neural network training with TPU/GPU support.
3. Financial Modeling
- Create versatile models for risk analysis or derivative pricing with seamless dataset handling.
4. Scientific Computing
- Implement higher-order PDE solvers and numerical methods.
- Optimize physical simulations like fluid dynamics with JIT support.
A Step-By-Step Guide to Loop Optimization with JAX
To integrate JAX into your Python workflow, follow these steps:
1. Install JAX:
Start by installing JAX via `pip install jax jaxlib`.
2. Set Up GPU/TPU Hardware (Optional):
Ensure that hardware accelerators are available and that JAX supports them.
3. Migrate Your NumPy Codebase:
Replace `numpy` imports with `jax.numpy` for immediate performance gains.
4. Implement JIT Optimization:
Identify redundancies in expensive loops and use `jax.jit` to optimize.
5. Test for Resilience:
Use debugging tools like `jax.grad` or `jax.jacrev` to verify outputs.
6. Profile Performance Gains:
Measure execution times and resource usage for optimized workflows.
Drive Efficiency with Modern Python Tools
Optimization is no longer a luxury. It’s a necessity in high-performance computing. Jax arange on loop carry techniques provides a straightforward yet powerful way to achieve significant gains.
With GPU/TPU acceleration, vectorized operations, and JIT compilation, JAX empowers developers to refine their workflows and focus on solving complex problems more effectively. Integrating JAX into your stack will undoubtedly transform how you write Python code, whether you’re building machine learning pipelines, scientific simulations, or financial models.
Build Smarter, Faster Code Today
Not sure where to begin? Explore JAX’s capabilities and see how it transforms your operations. Stay connected to our community of high-performance computing enthusiasts for tips, guides, and support!
Frequently Asked Questions about “Jax arange on loop carry”
Q1: How does JAX compare to NumPy?
While JAX is based on NumPy and supports similar functions, it offers features like JIT compilation, GPU/TPU acceleration, and automatic differentiation that are absent in NumPy. For performance-critical applications, JAX outperforms NumPy. arrange
Q2: What is JAX’s primary use case for `arrange ()`?
JAX’s `arrange ()` is commonly used to create to create arrays in numerical computations. It’s particularly useful in initializing large datasets for high-performance applications.
Q3: Can I run JAX without a GPU/TPU?
Yes, JAX runs fine on CPUs. However, to maximize its full potential, it’s recommended that JAX be run on GPUs or TPUs (e.g., via Google Cloud).
Q4: Is JAX only for machine learning?
Not at all. While JAX excels in machine learning, it’s a versatile library suitable for high-performance numerical computation, including scientific simulations, optimization problems, and data analysis.
Q5: What are the biggest benefits of JIT compilation?
JIT compilation translates your Python functions into optimized machine code. This results in significantly faster execution, especially for operations involving loops or large data arrays.