Fashion
PyTorch Fashion MNIST Training Job Example with Estimator: A Step-by-Step Guide
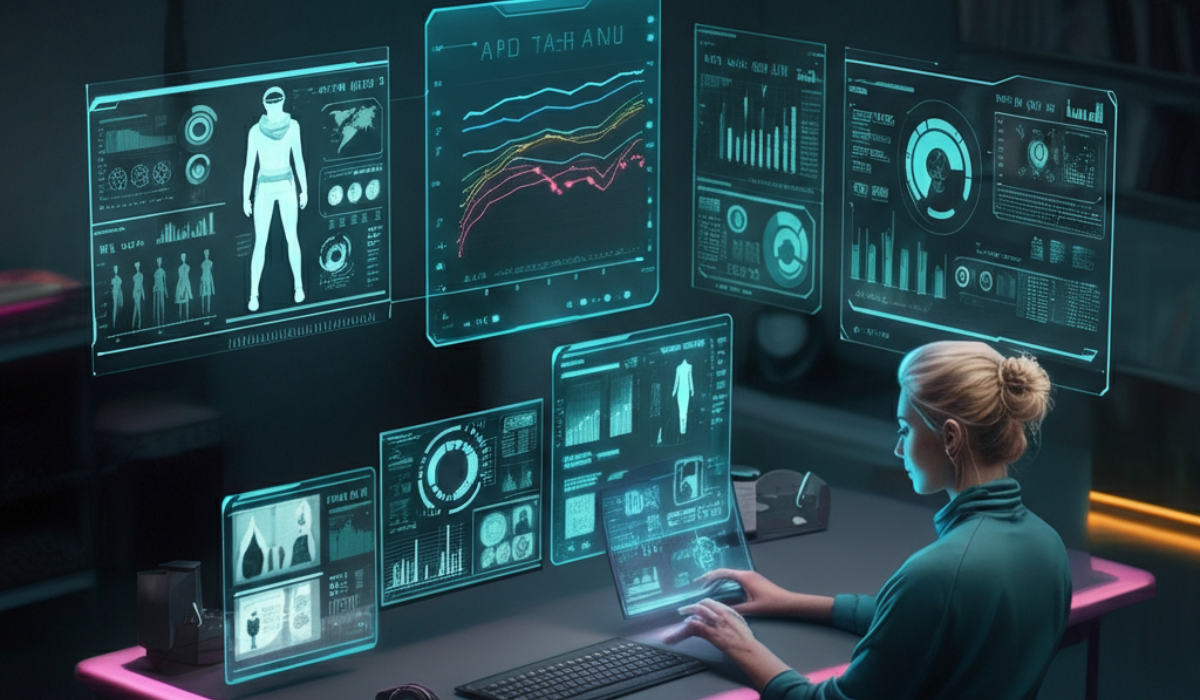
Deep learning and artificial intelligence have revolutionized industries ranging from healthcare to retail. Among the many deep learning frameworks available, PyTorch continues to stand out because of its flexibility and scalable operations. One specific showcase of PyTorch’s power is training a Fashion MNIST model using an estimator, a streamlined approach for efficiently executing machine learning jobs. This blog will walk you through the basics of training a PyTorch model on the popular Fashion MNIST dataset using an estimator for this task.
Suppose you’re a fashion enthusiast intrigued by how machines are learning to recognize patterns in clothing, whether it’s classifying sneakers or identifying a trench coat. In that case, this post serves as an introduction and a practical guide.
By the end of this blog, you’ll better understand how PyTorch Fashion MNIST Training Job Example with Estimator comes and how training with an estimator simplifies the process.
What is the Fashion MNIST Dataset?
Before we discuss the implementation details, it’s important to understand the dataset we’ll be working with. Fashion MNIST is a dataset developed to replace the older and less challenging MNIST dataset, which consisted of handwritten digits. Instead of digits, Fashion MNIST contains grayscale images of 70,000 fashion-related items, divided into 60,000 training and 10,000 testing examples.
Each image in Fashion MNIST is 28×28 pixels and represents one of ten clothing categories, such as:
- T-shirts/tops
- Trousers
- Dresses
- Sneakers
- Ankle boots
This dataset is widely used in deep-learning exercises. It is an ideal candidate for training a PyTorch model due to its diverse real-world application in classifying fashion items.
Why Use PyTorch and Estimator Together?
PyTorch provides robust tools for developing, testing, and deploying machine learning and deep learning models. However, manually handling every training step or managing infrastructure for training jobs can be tedious, especially for large models.
Enter the estimator. Estimators are convenient interfaces to handle distributed training jobs, parameterization, and scaling without much manual intervention. They allow you to abstract away much of the setup while providing an efficient framework for training models on large datasets like Fashion MNIST.
Key Benefits of Using an Estimator
1. Simplified Training Process:
Estimators streamline running ML jobs with simple configurations.
2. Scalability:
They can quickly scale up to handle massive datasets or complex architectures.
3. Customizability:
Estimators provide options for fine-tuning and adapting well to various deep-learning tasks.
4. Improved Deployment:
Post-training processes like fine-tuning and deployment are straightforward via estimator pipelines.
These benefits make estimators preferred for adopting a more modular and hassle-free workflow in PyTorch.
How to Train a PyTorch Fashion MNIST Model Using an Estimator
Here’s a multi-stage process to train the Fashion MNIST model effectively. We’ll break it down into the following steps:
Step 1. Import Libraries and Define Dependencies
To get started, we must import PyTorch and its essential dependencies.
Here is some sample code:
“`
import torch
from torch import nn, optim
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
from sagemaker.pytorch import PyTorch
“`
We’re using several libraries:
- `torch` for building and training the model.
- `torchvision` for accessing and transforming the Fashion MNIST dataset.
- `sagemaker.pytorch.PyTorch` to leverage an estimator for distributed model training.
Step 2. Preprocess the Dataset
Preprocessing involves normalizing and loading the dataset. The goal is to ensure the data is formatted and ready for the model.
Here’s how we do it:
“`
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,))
])
train_dataset = datasets.FashionMNIST(root=’./data’, train=True, transform=transform, download=True)
test_dataset = datasets.FashionMNIST(root=’./data’, train=False, transform=transform, download=True)
train_loader = DataLoader(train_dataset, batch_size=32, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=32, shuffle=False)
“`
The applied normalization ensures consistency in image grayscale values, a standard step in image preprocessing pipelines.
Step 3. Define the Model Architecture
Next, we build a basic convolutional neural network (CNN) to classify the Fashion MNIST images.
“`
class FashionMNISTModel(nn.Module):
def init(self):
super(FashionMNISTModel, self).init()
self.conv1 = nn.Conv2d(1, 32, kernel_size=3)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3)
self.fc1 = nn.Linear(6466, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = torch.relu(self.conv1(x))
x = torch.relu(self.conv2(x))
x = torch.flatten(x, 1)
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
“`
This architecture is simple yet effective, with layers for feature extraction (convolutional layers) and classification (fully connected layers).
Step 4. Configure and Train the Model with the Estimator
Once the model is ready, the estimator manages and executes the training job.
“`
estimator = PyTorch(entry_point=’fashion_mnist_training.py’,
role=’YOUR_AWS_ROLE’,
instance_count=2,
instance_type=’ml.c4.xlarge’,
framework_version=’1.5.0′,
py_version=’py3′)
estimator.fit({‘training’: ‘s3://your_bucket/training’})
“`
Here’s what happens:
- `entry_point` specifies the Python script containing model training logic.
- `instance_type` and `instance_count` define the computational resources.
- `fit()` launches the training job using the specified dataset.
Step 5. Evaluate the Model
After training, evaluate the model’s performance on the test dataset.
“`
def evaluate(model, loader, criterion):
model.eval()
total_loss, correct = 0, 0
with torch.no_grad():
for images, labels in loader:
outputs = model(images)
loss = criterion(outputs, labels)
total_loss += loss.item()
predictions = torch.argmax(outputs, dim=1)
correct += (predictions == labels).sum().item()
accuracy = correct / len(loader.dataset)
print(f”Test Accuracy: {accuracy:.2f}”)
“`
This function calculates the accuracy, which indicates how well the model performs in classifying the items.
Step 6. Deploy the Model
Once the performance is satisfactory, deploy the model for inference. Estimators are incredibly useful here, as deployment configurations are quite adaptable.
“`
predictor = estimator.deploy(initial_instance_count=1, instance_type=’ml.m5.large’)
“`
This command creates a live endpoint to serve predictions for incoming data.
Final Thought
Training a PyTorch model on the Fashion MNIST dataset using an estimator offers substantial scaling benefits. By combining PyTorch’s powerful features with estimators’ efficiency, you can build robust solutions that achieve high levels of accuracy while saving development time.
Are you ready to build your own models and work with datasets like Fashion MNIST? Whether you’re a data scientist or a fashion enthusiast fascinated by AI’s applications, PyTorch provides a modular, user-friendly toolkit to bring your ideas to life.
Give it a try today and experience how deep learning shapes the future of industries!
FAQs about PyTorch Fashion MNIST Training Job Example with Estimator
1. What is the main purpose of Fashion MNIST?
The Fashion MNIST dataset offers an entry point for training and testing AI models on more realistic and meaningful data than the MNIST digit dataset.
2. Why is PyTorch preferred over TensorFlow for beginners?
PyTorch offers a more Pythonic experience with transparent debugging and dynamic graph creation, making it friendlier for new developers.
3. Can Estimators handle large-scale distributed training?
Yes, Estimators abstract the complexities of managing distributed training across GPUs or multiple devices.
4. What categories are included in the Fashion MNIST dataset?
Fashion MNIST has 10 categories, including T-shirts, trousers, pullovers, dresses, coats, sandals, shirts, sneakers, bags, and ankle boots.
5. How do I optimize the model for faster training?
Experiment with techniques like learning rate scheduling, gradient clipping, and transferring the training process to a GPU.